Unser Bad Renovierungsprojekt dauert länger als gedacht. Immerhin sind wir heute voran gekommen. Die letzten 2,5 Wochen mussten wir vor allem auf das Trocknen von Wand und Boden warten. Heute war Abdichten dran. Morgen wird gefliest!
Port Askaig im Whisky Sour 🥃 😍
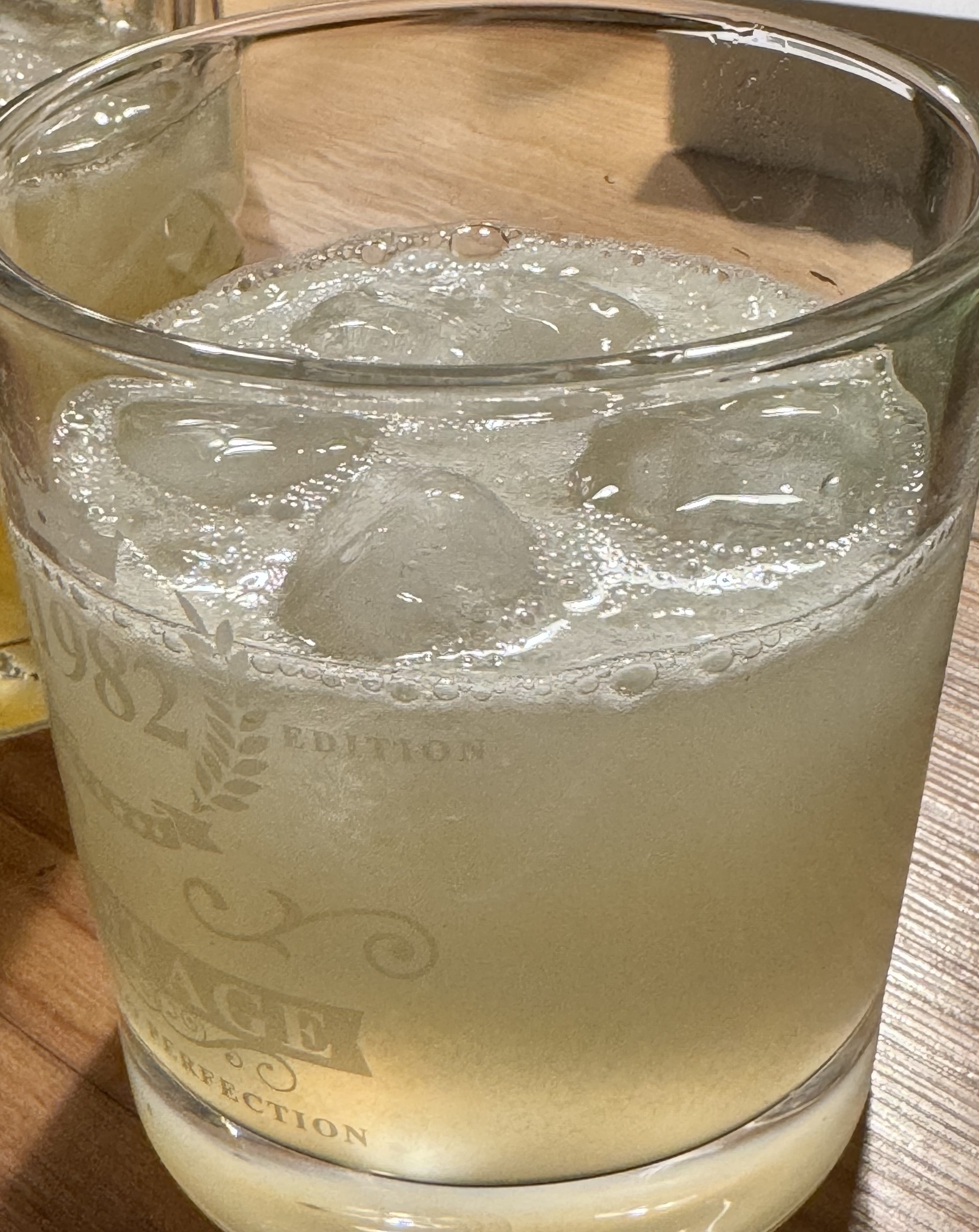
Bin den halben Weg zur Arbeit gelaufen. Der Herbst ist da.
On my way to the barbershop yesterday. I liked how there’s such a clear vantage point.
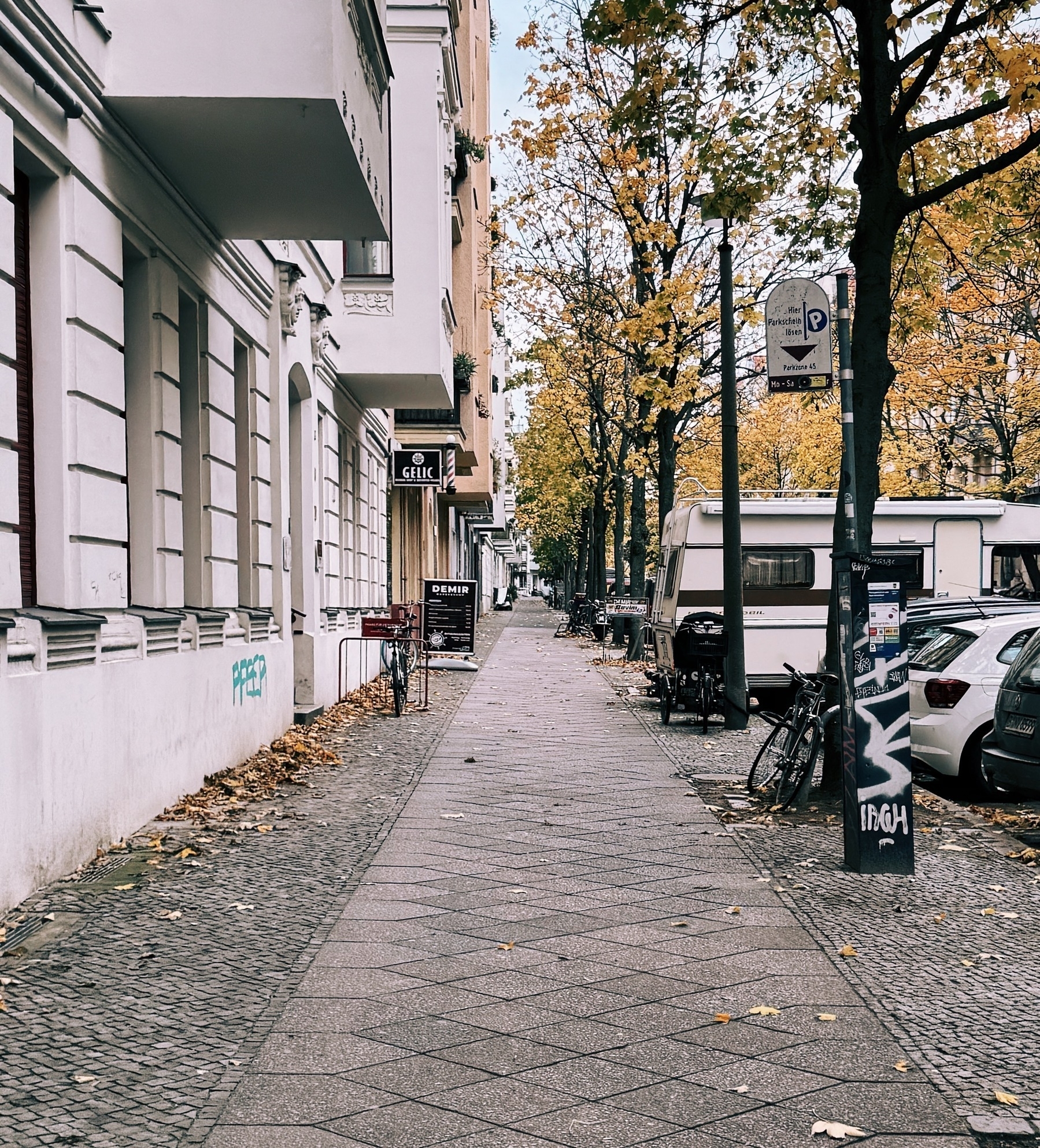
Guter Artikel!
Debatte über Migration: Warmes Herz, kühler Kopf - taz.de
Wir müssen uns ehrlich machen: Wir schaffen das nicht mehr. Es kommen zu viele Menschen zu uns, von denen sehr viele keine Bleiberechtsperspektive haben. Der Artikel 16a im Grundgesetz ist Auftrag und Verpflichtung zugleich. Wer unter dessen Schutzbereich fällt, dem wollen und dem müssen wir als Kommune helfen und Asyl gewähren, dies ist unstrittig. Wir müssen aber unterscheiden zwischen Asylbewerbern, Kriegs- und Katastrophenflüchtlingen und Menschen, die aus […] wirtschaftlichen Gründen zu uns kommen.
I was 94% accurate! ✅⬜️⬜️✅✅✅✅✅ Can you do better? www.brilliant.org/challenge…
Great game!
We did a little walk through central Berlin on the weekend.
My current desk setup. Went back to one monitor + laptop screen.
Finished reading: Tomorrow, and Tomorrow, and Tomorrow by Gabrielle Zevin 📚 I liked this a lot. Gamers and people born in the late 70s/early 80s have much to relate to.
This is new (to me) in Apple Music. Animations!
Das war der Beginn…
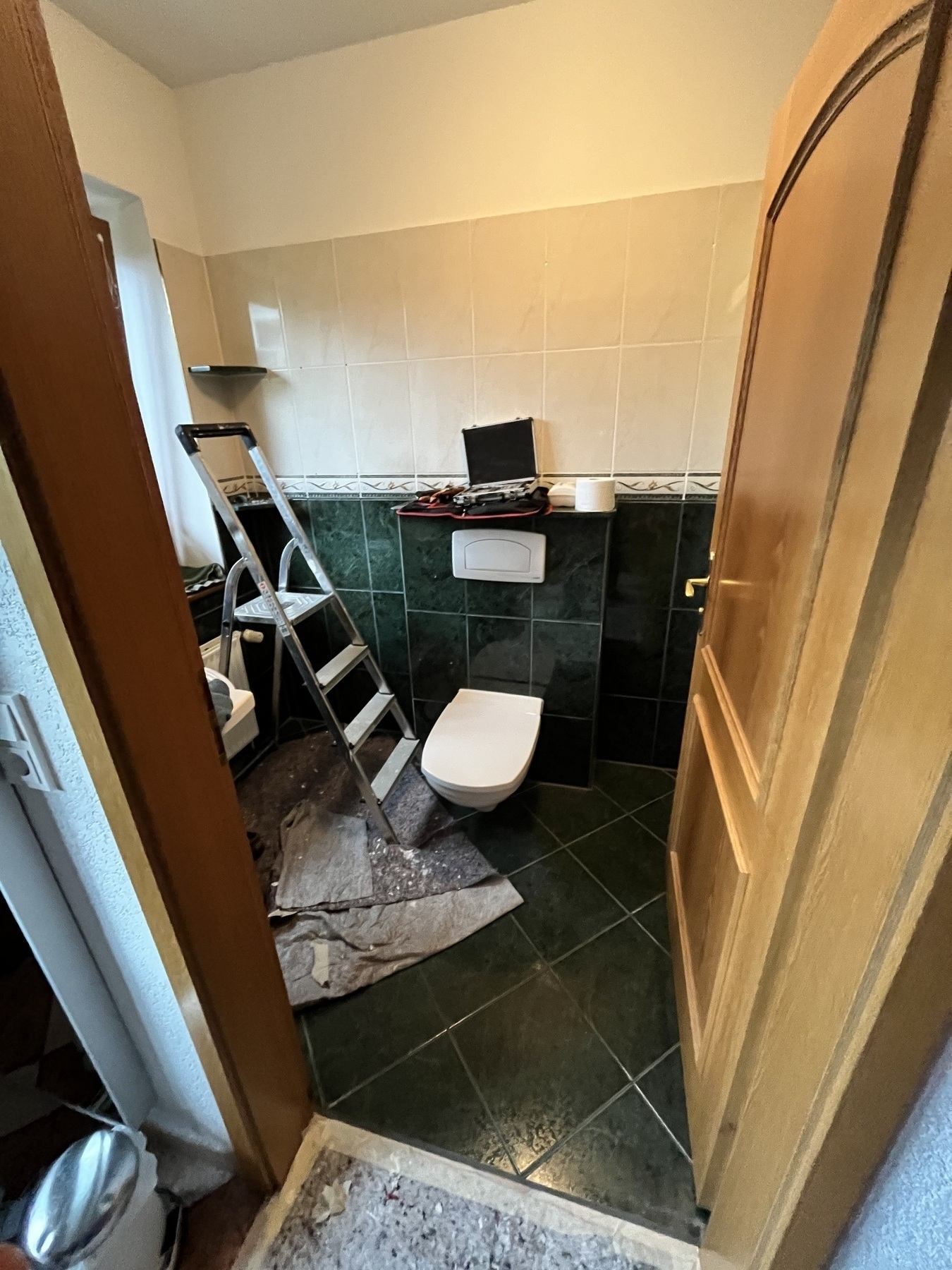
So sehen vier Stunden Arbeit aus. Gut voran gekommen, aber noch viel zu tun.
Eben waren wir im Baumarkt und haben die Fliesen abgeholt. Außerdem Fliesenkleber und anderen Kram. Jetzt leerräumen und vorbereiten. Nachher werden die ersten Fliesen fallen. 🏗️👷♂️💪
Dev note for catalog 2023-10-20
What I was able to achieve
Today I continued work on the catalog. I streamed for about 90 minutes to Twitch while working. I continued work where I had left it the last time which were the tests for the route /entries
. The goal was to get a passing test where the entries are rendered with their titles.
It took me longer than I care to admit but in the end I noticed my error and was able to get a passing test.
Next I talked a bit about the architecture decision records that I use to document my decisions on how to build things. I used a new ADR to document my decision about integrating the Pinboard.in API. Since a lot of my entries will come from Pinboard, I wanted to include the API access early on. That way I am able to get content to display “quickly”. At least that was the plan. I decided against using an existing Gem for Pinboard and will write my own API wrapper. While reading the docs for Pinboard I noticed that there is a new API v2 in development. I will use that new version.
I had to quit the session just as I was about to make a successful authenticated API call. Since I develop in a test-driven development style I had written a test that tests for the unauthenticated access. That test is successful in checking the 403
return code of the response. The successful/authenticated test case is not successful, yet. This is were I will continue next time.
My plan is to get started with that rather quickly, and then fetch entries from Pinboard and display them on the site.
About the streaming
I believe no one watched. I am not sure, Twitch told there was one visitor, but I believe that was I, monitoring the stream and watching for chat messages. So yeah, speaking into the void — but it was fun. I definitely need to improve my stream game. Switching between the VSCode and browser window is annoying. Don’t know how to do it better, yet. I will try to watch some other software development streams to get some inspiration how they do it. And my VSCode was probably too small to read. Well, next time!
Starting my stream now. Be gentle I am a beginner with streaming twitch.tv/holgerfro…
Really happy that I’ve discovered Lofi Girl for background music. Their streams really hit my mood on most days, depending on what I need. Jazzy vibes or more electro thingy. Today it’s Synthwave Radio
I went to YouTube with the goal of finding and watching a video for a specific topic/question. Then I saw thumbnails of other videos and clicked. 20 minutes later I returned and completely forgot about what I wanted to watch & learn about in the first place. 🤦♂️ Hello brain. Time to wake up.
Developing React Native apps really is a major PITA.
I use Macs since ~2008. I work as a professional programmer since ~2011. Since then I wasn’t able to use AppleScript (and recently Shortcuts.app) to automate my Mac. I don’t know whether this is due to me being stupid or because AppleScript and Shortcut syntax are really strange.
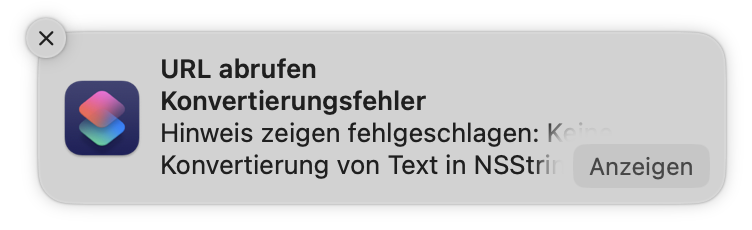
I hate computers. Maybe I should become a baker.